In this lesson I shall introduce several functions and show you actual OpenGLrendering in a program. Prior to showing you the code, however, I want to goover a few things with you. This will give you a better understanding of whatis going on when you do see the code, so you don't stare at the screenwondering what you're looking at. So, on with the show.
- Opengl Paint Program Source Code Generator
- Opengl Paint Program Source Code Free
- Opengl Paint Program Source Code Download
- Opengl Paint Program Source Code
New to OpenGL, working on “paint” program. I'm taking a computer graphics course this semester at college and our first assignment is to build a program that works much like Microsoft paint. We need to set options for drawing with shapes of different colors, sizes, and transparency parameters.
Transformations in OpenGL rely on the matrix for all mathematical computations. No, not the movie. Concentrate grasshopper. OpenGL has what is known as a matrix stack, which comes in handy for constructing models composed of many simple objects.
- The quality and speed of rendering in the GLWidget depends on the level of support for multisampling and hardware acceleration that your system's OpenGL driver provides. If support for either of these is lacking, the driver may fall back on a software renderer that may trade quality for speed. Example project @ code.qt.io.
- Accept Solution Reject Solution. Using GLUT, the following code will do this: (you may need to aquire glut or freeglut to be able to compile this. The alternative, just using a frame/dialog based app and your own code to setup the window, process the keys, handle idle times, handle resizing etc, etc is quite long-winded.
- The Open Toolkit library is a fast, low-level C# wrapper for OpenGL, OpenAL & OpenCL. It also includes windowing, mouse, keyboard and joystick input and a robust and fast math library, giving you everything you need to write your own renderer or game engine. OpenTK can be used standalone or inside a GUI on Windows, Linux, Mac.
The modelview matrix defines the coordinate system that is being used to place and orient objects. It is a 4x4 matrix that is multiplied by vertices and transformations to create a new matrix that reflects the result of any transformations that have been applied to the vertices. When we want to modify the modelview matrix we use the command glMatrixMode(). We define this asBefore you call any transformation commands you MUST specify whether you want to modify the modelview matrix or the projection matrix. The argument for modifying the modelview matrix is GL_MODELVIEW. So the complete line would appear as:Now we will look at translation. Translation allows you to move an object from one location to another within a 3D environment. The functions for this in OpenGL are glTranslatef() and glTranslated(). Here are their descriptions:Note that you must pass float types to glTranslatef() and double types to glTranslated(). X, Y, and Z represent the amount of translation on that axis.
Rotation in OpenGL is accomplished through the glRotate*() function, which is defined asNow let's take a look at these, and a few others, functions mentioned in a program. The following code is taken from OpenGL Game Programming and is commented by myself. If you have any problems building and using this code, feel free to contact me.That's a lot of code! Spend some time studying the example, practice a bit, and then we will proceed to lesson 7, where more of this code will be explained. Also, in lesson 7 we will cover Projections.Happy Coding!!
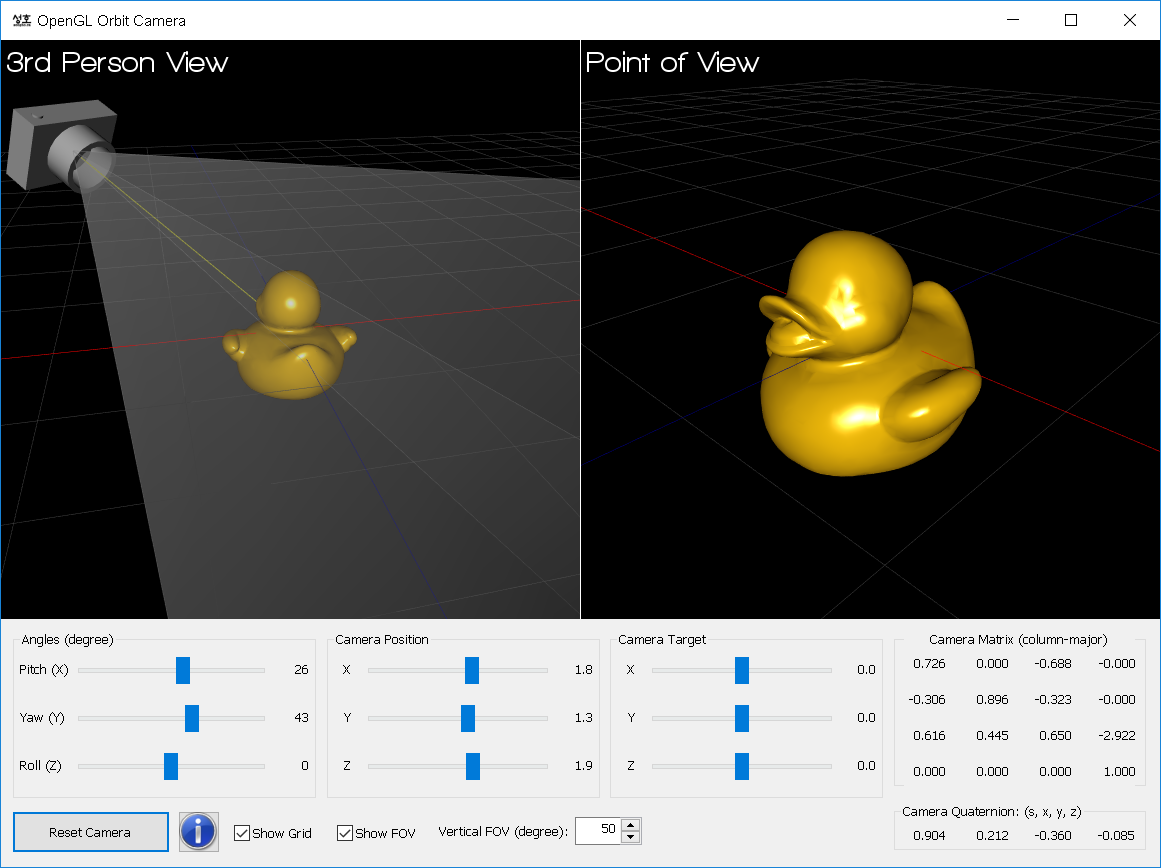
Previous: WGL functions
Next: Projections in OpenGL
Back to OpenGL tutorial index
Using the triangle geometry from OpenGL. But instead of using a mechanism such as glColor3d, use a pixel shader for each pixel in the triangle. The pixel shader should pick a random RGB color for each pixel. Most pixels should have colors which are different from that of most other pixels.
Optional: provide an update mechanism, to repeatedly re-render the triangle. (Possibilities include a mouse event handler, a timer event handler or an infinite loop, or even window expose events.) Shader generated color for each pixel should be different in each render.
Optional: after updating the opengl rendering target but before rendering the triangle, query the opengl implementation to determine which versions of shaders are supported by the rendering target, list the tested shaders and the available shaders and then use a supported shader.
See also: opengl.org's gl shader language documentation, and lighthouse3d.com's glsl tutorial.
C[edit]
Getting a true (pseudo) random number is surprisingly tricky. The following code makes something noisy, but not at all random:Go[edit]
The following uses 'cgo' to bind to the above C libraries. As C macro functions cannot be invoked directly from Go code, it has been necessary to wrap them first in 'normal' C functions and then invoke those.
JavaScript (WebGL)[edit]
Kotlin[edit]
Assuming that freeglut and GLEW are already installed on your system in the default location(s), you first need to build opengl2.klib using the following .def file and the cinterop tool:
You then need to compile the following Kotlin program, linking against opengl2.klib, and run the resulting .kexe file to view the rotating triangle.
Ol[edit]
Opengl Paint Program Source Code Generator
Phix[edit]
Note that several routines added for this demo have not yet added to the documentation, as you can tell from the syntax colouring.
Likewise no attempt has yet been made to make this run under pwa/p2js.
Opengl Paint Program Source Code Free
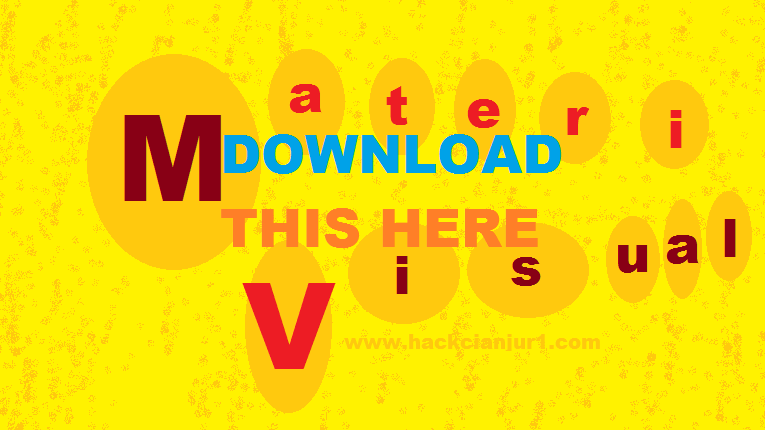
Racket[edit]
Tcl[edit]
Opengl Paint Program Source Code Download
Using the Tcl3d library and liberally borrowing from this pixel shader demo on the wiki, here is a brute translation of the C implementation.